Its very common and good practice to use a configuration file if you want your application to be externally configurable. This article demonstrates how to create and use one in your C# Console Application.
First, if your project don’t already have one, you can add an App.config file to your Console Project. If you are using Visual Studio, right-click on the project’s name in the solution explorer, then select Add > New Item…
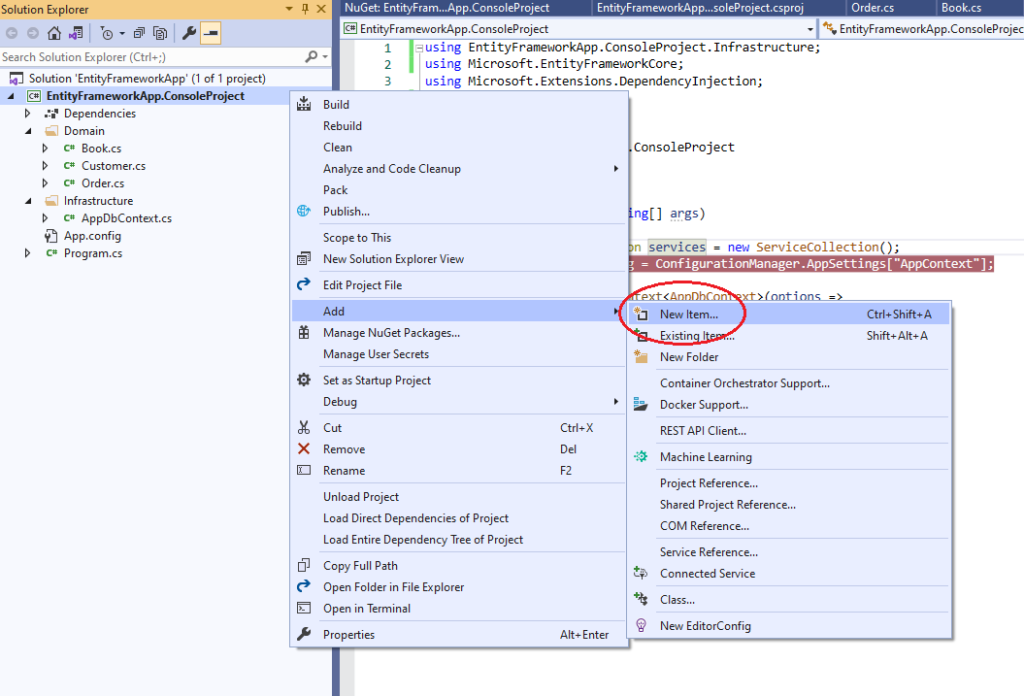
Next, type ‘config’ in the search box, select the result ‘Application Configuration File‘ and click Add.
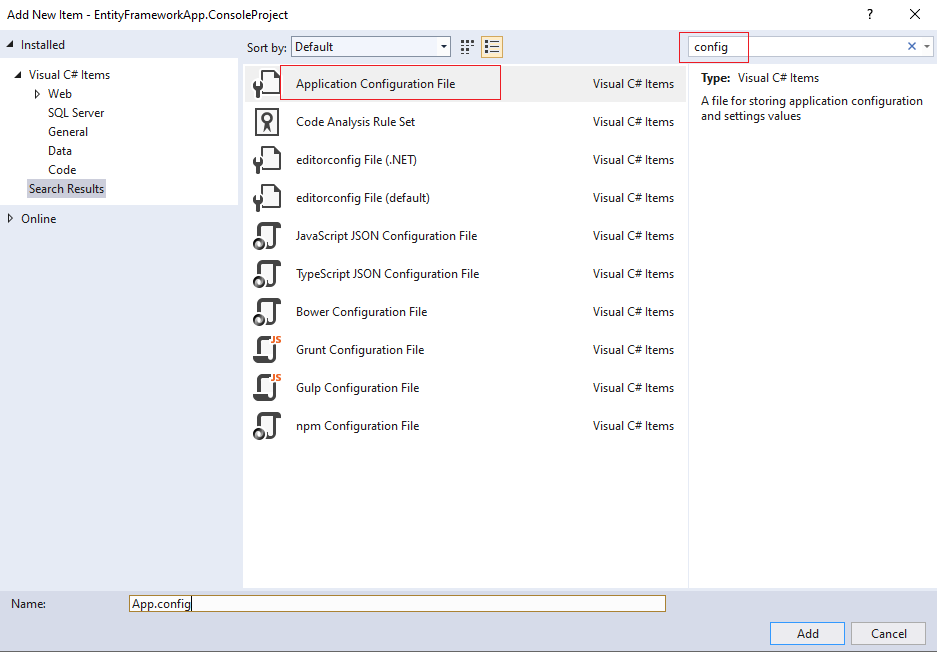
The new App.config file should now be visible in your project in the solution explorer.
Now, we can add a new entry with a key VariableName and value 100 in it:
<?xml version="1.0" encoding="utf-8" ?>
<configuration>
<appSettings>
<add key="VariableName" value="100" />
</appSettings>
</configuration>
As shown above, the App.config file is structured XML format.
Using Settings from App.Config in Code
To access the values we’ve added inside the App.config file, we need to install the System.Configuration.ConfigurationManager package. You can add this package via NuGet Package Manager in Visual Studio or by adding the following entry in the csproj file ( in .NET5 or higher, you can open this file by double-clicking the project in the solution explorer).
<Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<OutputType>Exe</OutputType>
<TargetFramework>.net5</TargetFramework>
</PropertyGroup>
<ItemGroup>
<PackageReference Include="System.Configuration.ConfigurationManager" Version="5.0.0" />
</ItemGroup>
</Project>
Finally, in the Main method, we can use the ConfigurationManager class to read any property we want from the App.config:
class Program
{
static void Main(string[] args)
{
string value = ConfigurationManager.AppSettings["VariableName"];
Console.WriteLine($"The value of property VariableName is {value}");
}
}
The output will be:
The value of property VariableName is 100