A particulate sensor is a device that detects particles in the air and measures their concentration. These particles can be dust, pollen, car emissions, vapors from liquids, and more. With such sensors, you can build systems that monitor air quality. One example is using these sensors to track emissions from 3D printers.
How PMS5003 Works
The PMS5003, like many other particulate sensors, is a laser-based dust sensor. It uses the principle of laser light scattering to measure the amount of dust particles suspended in the air. This gives us an indication of how polluted the air is.
The PMS5003 sensor detects three types of particulate matter (PM1, PM2.5, PM10):
PM1 | Consists of ultra-fine particles with a diameter smaller than 1 micron (0.1) |
PM2.5 | Often referred to as fine particulate matter, includes particles with a diameter under 2.5 microns. |
PM10 | Includes particles measuring less than 10 microns in diameter, which is about 100 times smaller than a millimeter. |
3D Printer Emissions
Studies show that 3D printers emit ultrafine particles that are smaller than 100 nm (0.1μm). This size is near the edge of the PMS5003’s detection capabilities. To measure particles smaller than this, more expensive equipment would be required. However, the PMS5003 can still detect harmful particles in the range of 1μm to 10μm, which are common in 3D printer emissions.
PMS5003 PINOUT
We donât need to use all the pins on the sensor, just the essential ones. Below is the full pinout for the PMS5003 sensor:
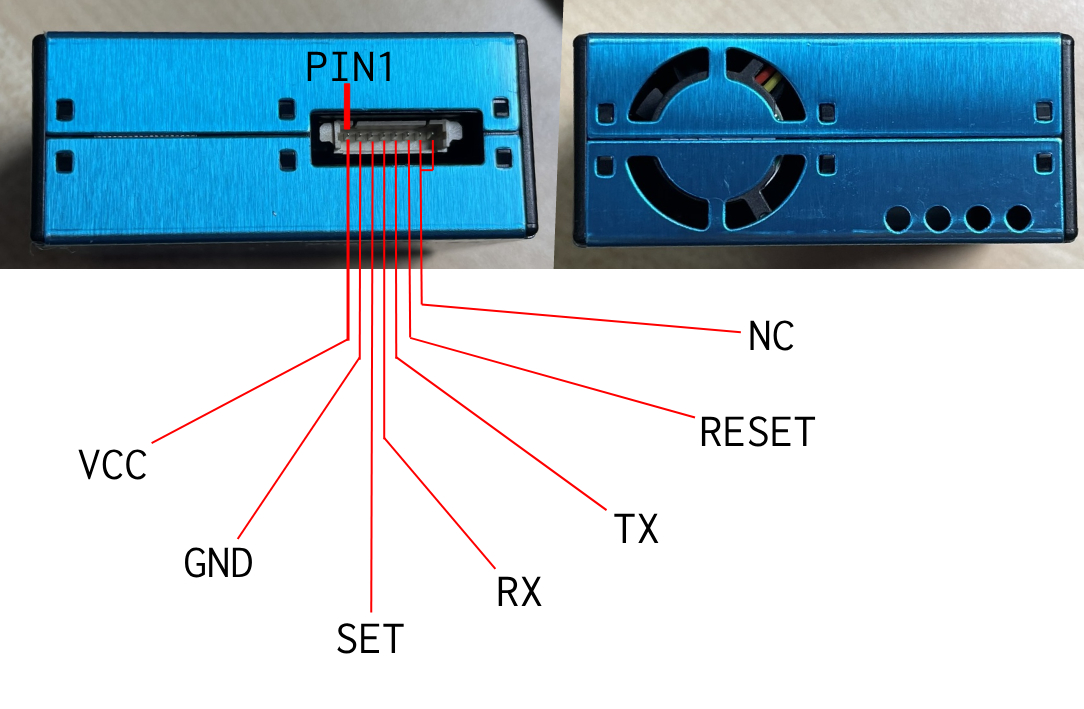
PIN1 | VCC | Positive power 5V |
PIN2 | GND | Negative power |
PIN3 | SET | Set pin /TTL level@3.3Vï¼high level or suspending is normal working status, while low level is sleeping mode. |
PIN4 | RX | Serial port receiving pin/TTL level@3.3V. Will be connected to the TX of the Arduino. |
PIN5 | TX | Serial port sending pin/TTL level@3.3V. Will be connected to the RX of the Arduino. |
PIN6 | RESET | Module reset signal /TTL level@3.3V, low reset. |
PIN7 | NC | No-Connect |
PIN8 | NC | No-Connect |
PMS5003 Arduino Circuit
The RX pin of the PMS5003 sensor is connected to the TX pin of the Arduino.
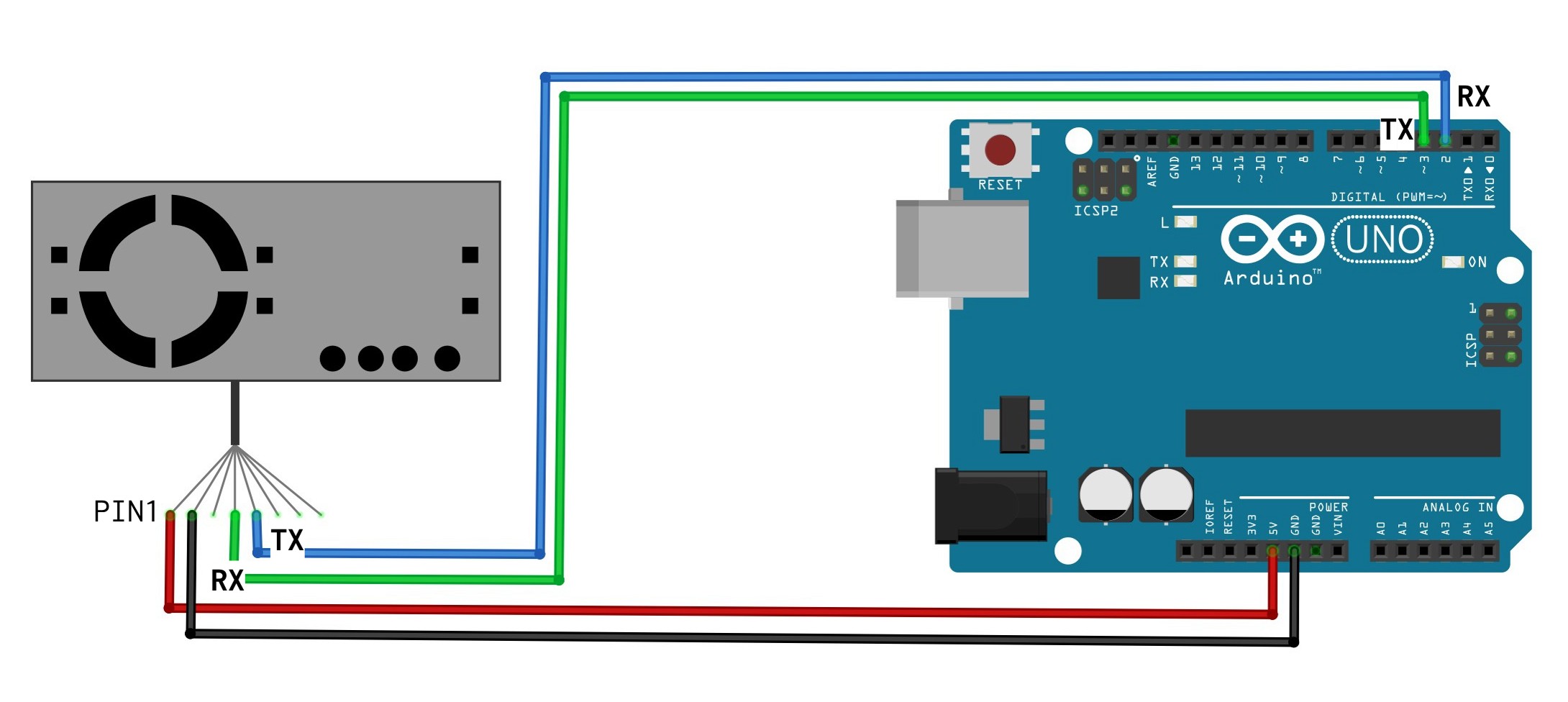
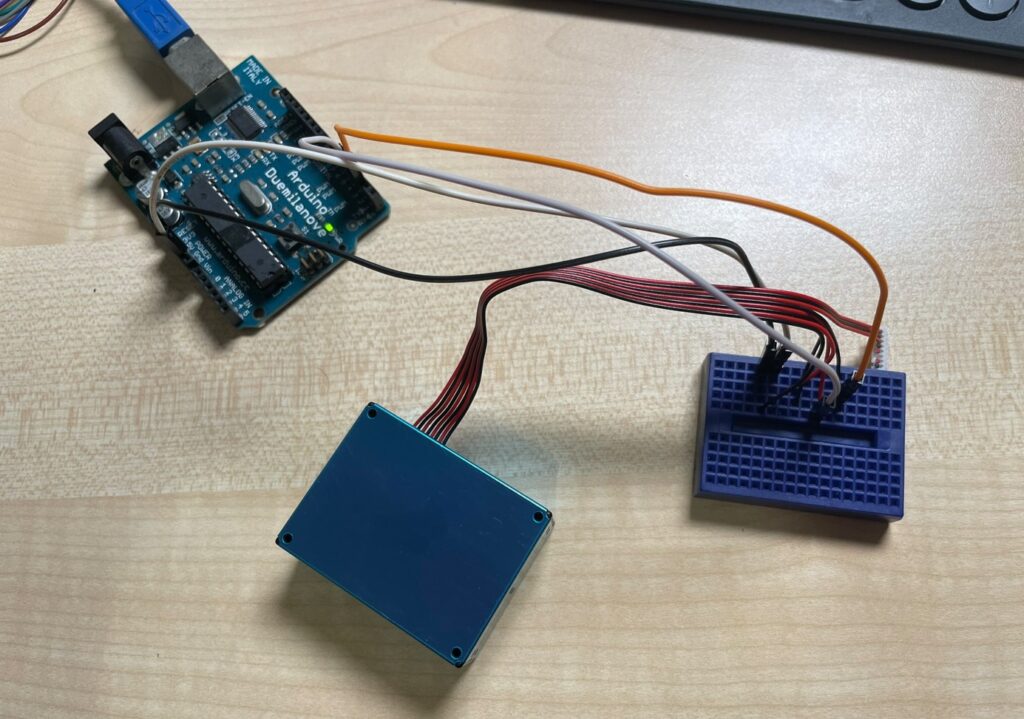
PMS5003 and Arduino Code
To begin using the PMS5003 sensor with Arduino, we need to import the correct library into the Arduino IDE. The PMS5003 sensor in this guide is from Plantower, so weâll use the relevant PMS Library as shown below:
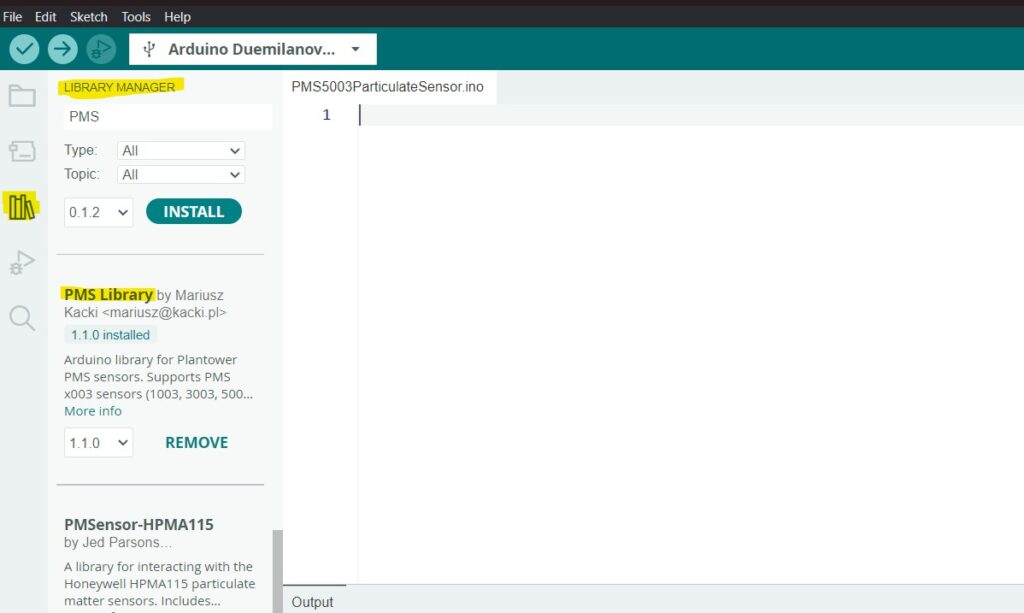
After importing the library, we can import the PMS.h library to the code.
Use the following code to interface with the sensor using SoftwareSerial. This allows us to assign two arbitrary pins on the Arduino for RX and TX communication with the sensor.
#include "PMS.h"
#include "SoftwareSerial.h"
SoftwareSerial Serial1(2, 3); // RX, TX
PMS pms(Serial1);
PMS::DATA data;
void setup()
{
Serial.begin(9600);
Serial1.begin(9600);
delay(4000);
}
void loop()
{
if (pms.read(data))
{
Serial.println("PM1.0: " + String(data.PM_AE_UG_1_0) + "(ug/m3)");
Serial.println("PM2.5 : " + String(data.PM_AE_UG_2_5) + "(ug/m3)");
Serial.println("PM10 : " + String(data.PM_AE_UG_10_0) + "(ug/m3)");
Serial.println("--------------------------------------------");
delay(1000);
}
}
When the sensor is running, it will output the concentrations of PM1.0, PM2.5, and PM10 particles. These values will be shown on the Serial Monitor in the Arduino IDE, as shown below:
PM1.0: 1(ug/m3)
PM2.5 : 4(ug/m3)
PM10 : 4(ug/m3)
--------------------------------------------
PM1.0: 1(ug/m3)
PM2.5 : 4(ug/m3)
PM10 : 4(ug/m3)
--------------------------------------------
PM1.0: 1(ug/m3)
PM2.5 : 4(ug/m3)
PM10 : 4(ug/m3)
--------------------------------------------
The Serial Monitor will display this information every second, allowing you to track air quality continuously.
Using a PMS5003 particulate sensor, you can easily measure the concentration of dust particles in the air. Whether youâre monitoring emissions from a 3D printer or just interested in air quality, this sensor provides useful data on particle pollution. By connecting it to an Arduino and reading the data through the Serial Monitor, you can build a simple but effective air quality monitoring system.